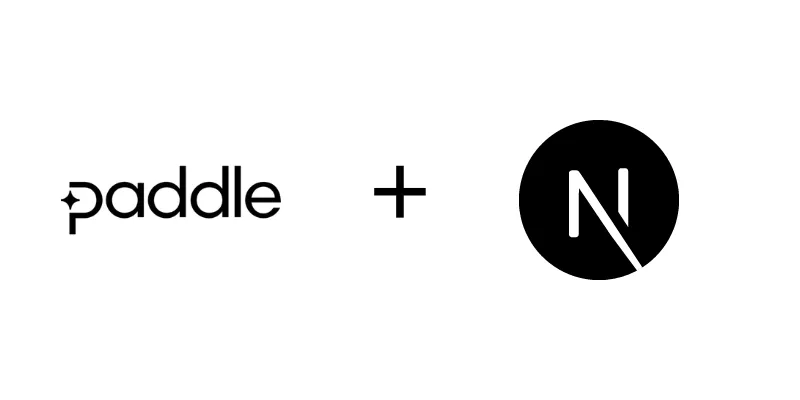
Why Paddle ?
Paddle is a not just a payment processor as stripe, it provides complete payment infrastructure. Paddle uses a merchant of record (MoR) model to provide an all-in-one payments, billing, and sales tax solution.
Paddle handles tax and remittance globally, taking full liability for sales tax, fighting fraud, chasing chargebacks, managing reconciliation and ensuring compliance along the way. All for
5% + 50¢
per transaction.
Prerequisites
Before you begin, you’ll need to have the following:
- Paddle sandbox account (sign up at Paddle)
- Next js 14 App
Setting up Environment Variables
PADDLE_ENV= "sandbox" or "production"
PADDLE_CLIENT_TOKEN=""
PADDLE_SELLER_ID=""
Find the Paddle Client Token and Seller ID in your Paddle Dashboard under Developer Tools > Authentication.
Install Paddle.js
First, install the Paddle.js package using a package manager.
pnpm add @paddle/paddlejs
It is the official package from Paddle, it contains typescript definitions as well.
Initializing Paddle
Now let’s initialize paddle in our project, for that we can create a hook called usePaddle.
// hooks/usePaddle.tsx
"use client";
import { initializePaddle, InitializePaddleOptions, Paddle } from "@paddle/paddle-js";
import { useEffect, useState } from "react";
export default function usePaddle() {
const [paddle, setPaddle] = useState<Paddle>();
useEffect(() => {
initializePaddle({
environment: process.env.PADDLE_ENV! ? "production" : "sandbox",
token: process.env.PADDLE_CLIENT_TOKEN!,
seller: Number(process.env.PADDLE_SELLER_ID),
} as unknown as InitializePaddleOptions).then((paddleInstance: Paddle | undefined) => {
if (paddleInstance) {
setPaddle(paddleInstance);
}
});
}, []);
return paddle;
}
Creating a Product catalog in Paddle Dashboard
You can create a product catalog in Paddle Dashboard under Catalog > Products. You can create a product with a name, description, price, and image. You can also create a subscription product with a trial period and country specific pricing.
Creating a Checkout Button
Now we can create a checkout button, for that we can create a component called CheckoutButton.
"use client"
import usePaddle from "../hooks/usePaddle";
export default function CheckoutButton(){
const paddle = usePaddle();
const openCheckout = () => {
paddle?.Checkout.open({
items: [
{
priceId: "pri_1234567890", // you can find it in the product catalog
quantity: 1,
},
],
customer: {
email: "customer@email.com" // email of your current logged in user
},
customData: {
// other custom metadata you want to pass
},
settings: {
// settings like successUrl and theme
}
});
};
return (
<button
onClick={openCheckout}
>
Checkout
</button>
);
}
Continue checkout flow
Now you can run the dev server and test the checkout. It will work fine, the transaction will be shown to you in the Paddle dashboard. But for handling the events during the checkout flow, we need to use Paddle webhook so that we can make changes to the database according to the occurrence of events.
We will learn more about the webhook and its usage in next 14 in next blog post.
- niraj